The following article used to create a remote service
1.Create a Project Called RSDemo. 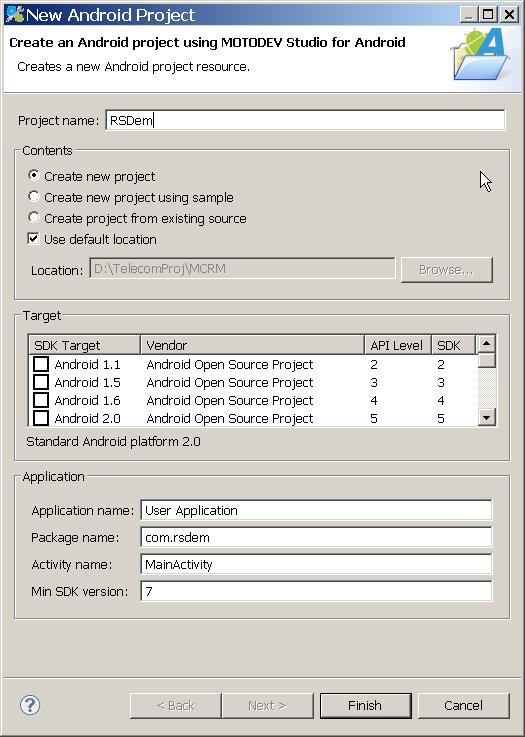
2.Create a aidl in your IDE :
AIDL (Android Interface Definition Language) is an
IDLlanguage used to generate code that enables two processes on an Android-powered device
to talk using interprocess communication (IPC). If you have code
in one process (for example, in an Activity) that needs to call methods on an
object in another process (for example, a Service), you would use AIDL to
generate code to marshall the parameters.
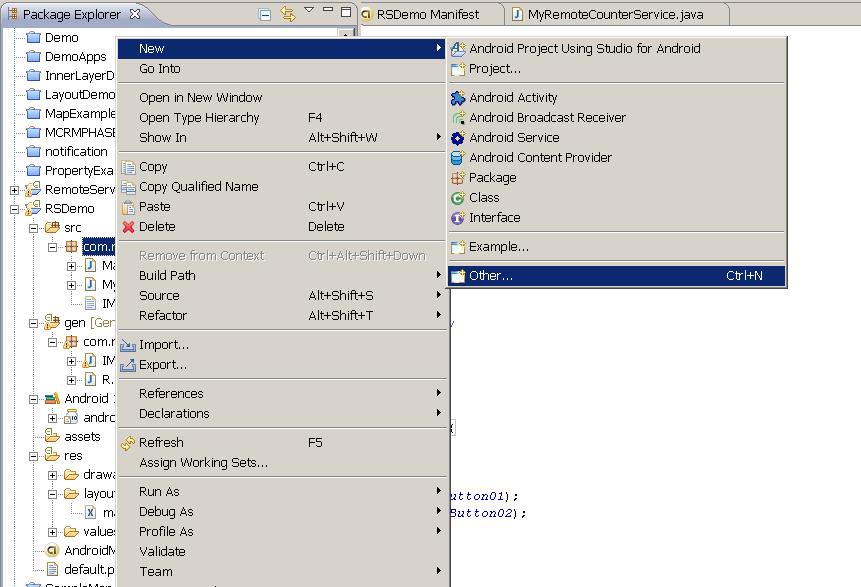
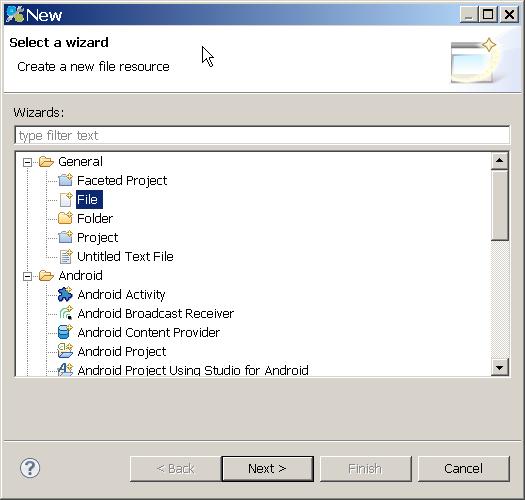
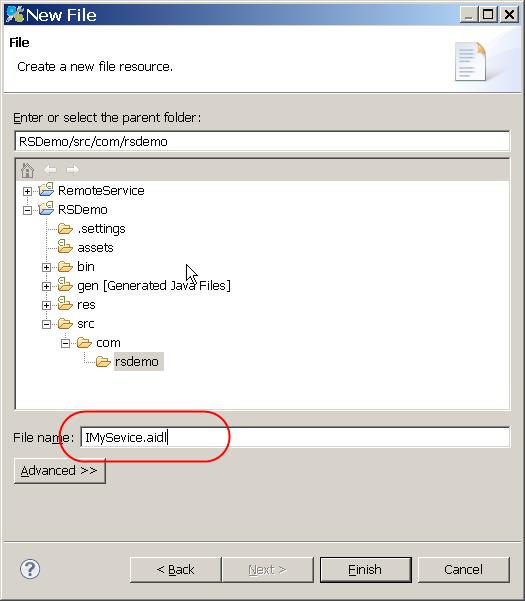
AIDL is a simple syntax that lets you declare an interface with one or more methods, that can take parameters and return values. These parameters and return values can be of any type, even other AIDL-generated interfaces. However, it is important to note that you must import all non-built-in types, even if they are defined in the same package as your interface.
Sample AIDL File package <<<YOUR PROJECT PACKAGE >>>;
interface IMyService {
int getStatus();
}
3. Ensure the Stub file in gen Folder.
4. Create Service and create an instance for the stub class
Create a Service Class
Create a instance for stub class with implmentation
Return the instance while calling the IBind Method.
public class MyRemoteCounterService extends Service {
/**
* @see android.app.Service#onBind(Intent)
*/
private IMyService.Stub imyserviceStub = new IMyService.Stub() {
@Override
public int getStatus() throws RemoteException {
return 1;
}
};
@Override
public IBinder onBind(Intent intent) {
Log.d("REMOTE SERVICE ", "INVOKED");
return imyserviceStub;
}
@Override
public void onCreate() {
super.onCreate();
}
@Override
public void onStart(Intent intent, int startId) {
super.onStart(intent, startId);
}
@Override
public void onDestroy() {
super.onDestroy();
}
}
5. Create a Client Class Access the Service. 1.Create instances for Button for invoking and release the Connection
2. Create a class Service Connector using the ServiceConnector Interface.
3. Create a Instance for SeviceConnector and Stub class
4. Bind the service using the bindService()
5. Invoke the Service
Create a class Service Connector using the ServiceConnector Interface class MyServiceStatus implements ServiceConnection {
@Override
public void onServiceConnected(ComponentName arg0,
IBinder arg1) {
iMyService = IMyService.Stub.asInterface((IBinder)arg1);
Log.d("@G RemoteService","Connected");
}
@Override
public void onServiceDisconnected(ComponentName name) {
iMyService = null;
Log.d("@G RemoteService","DisConnected");
}
}
Create a Instance for SeviceConnector and Stub class ivate IMyService iMyService;
private MyServiceStatus conn;
Bind the service using the bindService() if (conn == null) {
conn = new MyServiceStatus();
Intent i = new Intent();
i.setClassName("com.rsdemo", "com.rsdemo.MyRemoteCounterService");
bindService(i, conn, Context.BIND_AUTO_CREATE);
}
Invoke the Service try {
counter = iMyService.getStatus();
} catch (RemoteException re) {
Log.e(getClass().getSimpleName(), "RemoteException");
}
6. Sample Source
1. AIDL Implementation - IMyService.aidl
package com.rsdemo;
interface IMyService {
int getStatus();
}
Service - MyRemoteCounterService
public class MyRemoteCounterService extends Service {
/**
* @see android.app.Service#onBind(Intent)
*/
private IMyService.Stub imyserviceStub = new IMyService.Stub() {
@Override
public int getStatus() throws RemoteException {
return 1;
}
};
@Override
public IBinder onBind(Intent intent) {
Log.d("REMOTE SERVICE ", "INVOKED");
return imyserviceStub;
}
@Override
public void onCreate() {
super.onCreate();
}
@Override
public void onStart(Intent intent, int startId) {
super.onStart(intent, startId);
}
@Override
public void onDestroy() {
super.onDestroy();
}
}
Client
/** Called when the activity is first created. */
private IMyService iMyService;
private MyServiceStatus conn;
int counter = 0;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
Button invoke = (Button) findViewById(R.id.Button01);
Button release = (Button) findViewById(R.id.Button02);
if (conn == null) {
conn = new MyServiceStatus();
Intent i = new Intent();
i.setClassName("com.rsdemo", "com.rsdemo.MyRemoteCounterService");
bindService(i, conn, Context.BIND_AUTO_CREATE);
}
release.setOnClickListener(new OnClickListener() {
public void onClick(View v) {
unbindService(conn);
conn = null;
}
});
invoke.setOnClickListener(new OnClickListener() {
public void onClick(View v) {
if (conn == null) {
Toast.makeText(MainActivity.this,
"Cannot invoke - service not bound",
Toast.LENGTH_SHORT).show();
} else {
try {
counter = iMyService.getStatus();
} catch (RemoteException re) {
Log.e(getClass().getSimpleName(), "RemoteException");
}
}
Toast.makeText(MainActivity.this, counter + "",
Toast.LENGTH_LONG).show();
}
});
}
class MyServiceStatus implements ServiceConnection {
@Override
public void onServiceConnected(ComponentName arg0, IBinder arg1) {
iMyService = IMyService.Stub.asInterface((IBinder)arg1);
Log.d("@G RemoteService","Connected");
}
@Override
public void onServiceDisconnected(ComponentName name) {
iMyService = null;
Log.d("@G RemoteService","DisConnected");
}
}
4. Layout - main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical" android:layout_width="fill_parent"
android:layout_height="fill_parent">
<TextView android:layout_width="fill_parent"
android:layout_height="wrap_content" android:text="@string/hello" />
<Button android:id="@+id/Button01" android:layout_width="wrap_content"
android:layout_height="wrap_content" android:text="Invoke"></Button>
<Button android:id="@+id/Button02" android:layout_width="wrap_content"
android:layout_height="wrap_content" android:text="Release"></Button>
</LinearLayout>